You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
simplex noise in 3D with double precision integers produces a wrong noise patters that is not in the bounds of [-1,1].
here is an example that samples the noise at a coordinate where it is out of range. Additionally I create an image where you can see the difference between the float and the double version of the noise function.
#include<glm/glm.hpp>
#include<glm/gtc/noise.hpp>
#include<cstdlib>
#include<cstdio>usingnamespaceglm;usingnamespacestd;intmain() {
printf("%f\n", simplex( vec3(1.4, 2.7, 0.51))); // prints 0.438957printf("%f\n", simplex(dvec3(1.4, 2.7, 0.51))); // prints 3.93985constint dimx = 800, dimy = 800;
FILE *fp = fopen("noise-dvec3-error.ppm", "wb"); // b - binary modefprintf(fp, "P6\n%d %d\n255\n", dimx, dimy);
for (int j = 0; j < dimy; ++j) {
for (int i = 0; i < dimx; ++i) {
dvec3 pos(float(i) * 0.01, float(j) * 0.01, 0.51);
float noisef = simplex(vec3(pos));
double noised = simplex(pos);
unsignedchar a = (unsignedchar)(glm::clamp<float>((noisef * 0.5 + 0.5) * 256, 0.0f, 255.0f));
unsignedchar b = (unsignedchar)(glm::clamp<double>((noised * 0.5 + 0.5) * 256, 0.0d, 255.0d));
staticunsignedchar color[3];
color[0] = a;
color[1] = b;
// make out of range values more visibleif(b == 0 || b == 255)
color[2] = 255 - b;
else
color[2] = b;
(void) fwrite(color, 1, 3, fp);
}
}
fclose(fp);
return EXIT_SUCCESS;
}
Output Image:
red channel is the result of simplex(vec3)
green/blue channel is the result of simplex(dvec3)
blue channel is inverted if simplex(dvec3) needs clamping (is out of range)
A grayscale pixel means that simplex(vec3) and simplex(dvec3) as the same value and no clipping occurs
A colored pixel means simplex(vec3) and simplex(dvec3) disagrees on the result.
EDIT:
simplex of dvec4 does not even compile.
The text was updated successfully, but these errors were encountered:
auto value = glm::simplex(glm::dvec3 { 0.71805937418432031, -0.65074130785454032, -0.24683290987586010 });
// value == -1.0236474215821429, value not in [-1.0, 1.0],
simplex noise in 3D with double precision integers produces a wrong noise patters that is not in the bounds of
[-1,1]
.here is an example that samples the noise at a coordinate where it is out of range. Additionally I create an image where you can see the difference between the
float
and thedouble
version of the noise function.Output Image:
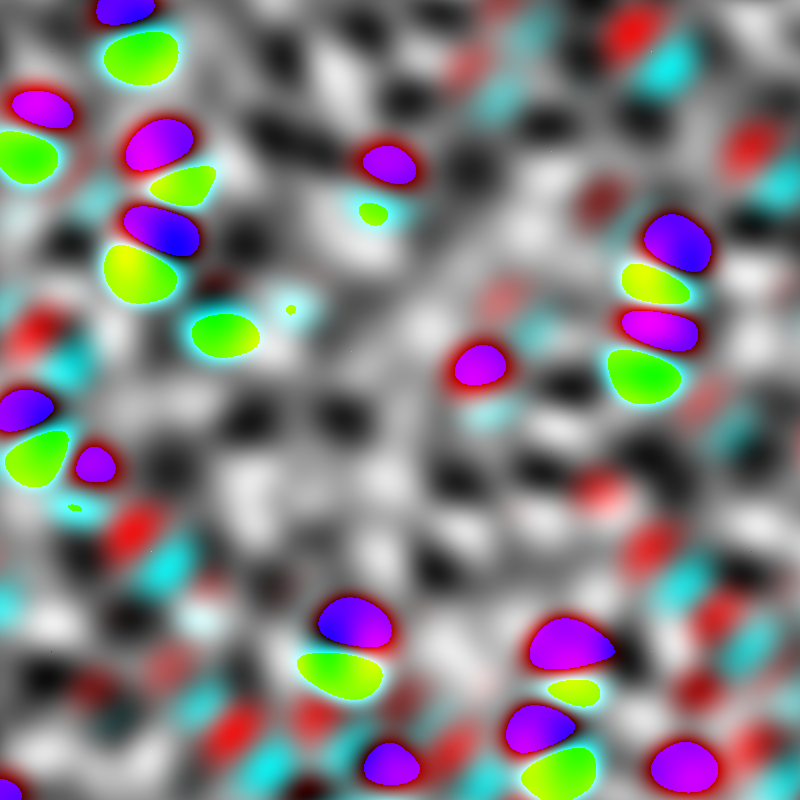
simplex(vec3)
simplex(dvec3)
simplex(dvec3)
needs clamping (is out of range)simplex(vec3)
andsimplex(dvec3)
as the same value and no clipping occurssimplex(vec3)
andsimplex(dvec3)
disagrees on the result.EDIT:
simplex of dvec4 does not even compile.
The text was updated successfully, but these errors were encountered: